ComboBox , Blazor 에서는 Select List 에 데이터를 바인딩하는 방법에 대해 설명합니다. Department
목록을 바인딩하려고 합니다. 모든 부서 목록을 바인딩하r고 해당 Employee 의 부서도 선택해야 합니다.
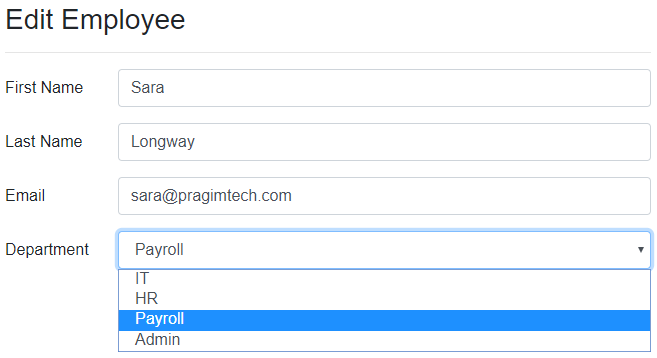
Blazor input select example
<InputSelect id="department" @bind-Value="DepartmentId"> @foreach (var dept in Departments) { <option value="@dept.DepartmentId">@dept.DepartmentName</option> } </InputSelect>
코드 설명
InputSelect component
를 사용하여 htmlselect
요소를 렌더링합니다.Departments
속성에 있는 모든 부서 목록을 화면에 표시합니다.foreach
로 부서목록을select
각 부서 요소를 만듭니다.- 옵션 값은 부서 ID이고 표시 텍스트는 부서 이름입니다.
- 직원이 IT 부서에 속해 있으면 선택해야 합니다. 이를 위해 @bind-Value 속성을 사용합니다. 이 속성은 양방향 데이터 바인딩을 제공합니다. 즉, 초기 페이지 로드 시 직원 부서가 선택되고 선택을 변경하면 새로 선택한 부서 값이
DepartmentId
구성 요소 클래스의 속성에 자동으로 전달됩니다. - 선택 요소를 정수에 바인딩하는 것은 지원되지 않으며 다음 예외를 throw합니다.
‘Microsoft.AspNetCore.Components.Forms.InputSelect`1[System.Int32] does not support the type ‘System.Int32”
Edit Employee Component View (EditEmployee.razor)
@page "/editemployee/{id}" @inherits EditEmployeeBase <EditForm Model="@Employee"> <h3>Edit Employee</h3> <hr /> <div class="form-group row"> <label for="firstName" class="col-sm-2 col-form-label"> First Name </label> <div class="col-sm-10"> <InputText id="firstName" class="form-control" placeholder="First Name" @bind-Value="Employee.FirstName" /> </div> </div> <div class="form-group row"> <label for="lastName" class="col-sm-2 col-form-label"> Last Name </label> <div class="col-sm-10"> <InputText id="lastName" class="form-control" placeholder="Last Name" @bind-Value="Employee.LastName" /> </div> </div> <div class="form-group row"> <label for="email" class="col-sm-2 col-form-label"> Email </label> <div class="col-sm-10"> <InputText id="email" class="form-control" placeholder="Email" @bind-Value="Employee.Email" /> </div> </div> <div class="form-group row"> <label for="department" class="col-sm-2 col-form-label"> Department </label> <div class="col-sm-10"> <InputSelect id="department" @bind-Value="DepartmentId" class="form-control"> @foreach (var dept in Departments) { <option value="@dept.DepartmentId">@dept.DepartmentName</option> } </InputSelect> </div> </div> </EditForm>
Edit Employee Component Class (EditEmployeeBase.cs)
using EmployeeManagement.Models; using EmployeeManagement.Web.Services; using Microsoft.AspNetCore.Components; using System.Collections.Generic; using System.Linq; using System.Threading.Tasks; namespace EmployeeManagement.Web.Pages { public class EditEmployeeBase : ComponentBase { [Inject] public IEmployeeService EmployeeService { get; set; } public Employee Employee { get; set; } = new Employee(); [Inject] public IDepartmentService DepartmentService { get; set; } public List<Department> Departments { get; set; } = new List<Department>(); public string DepartmentId { get; set; } [Parameter] public string Id { get; set; } protected async override Task OnInitializedAsync() { Employee = await EmployeeService.GetEmployee(int.Parse(Id)); Departments = (await DepartmentService.GetDepartments()).ToList(); DepartmentId = Employee.DepartmentId.ToString(); } } }
아래에서 REST API 를 사용하여 데이터베이스 테이블에서 부서 데이터를 검색하는 방법을 설명합니다.
REST API Project
IDepartmentRepository Interface
이 두 메서드는 데이터베이스 작업을 수행하며 작업을 반환하도록 비동기적으로 실행되기를 원합니다.
public interface IDepartmentRepository { Task<IEnumerable<Department>> GetDepartments(); Task<Department> GetDepartment(int departmentId); }
DepartmentRepository Class
public class DepartmentRepository : IDepartmentRepository { private readonly AppDbContext appDbContext; public DepartmentRepository(AppDbContext appDbContext) { this.appDbContext = appDbContext; } public async Task<Department> GetDepartment(int departmentId) { return await appDbContext.Departments .FirstOrDefaultAsync(d => d.DepartmentId == departmentId); } public async Task<IEnumerable<Department>> GetDepartments() { return await appDbContext.Departments.ToListAsync(); } }
Departments REST API Controller
[Route("api/[controller]")] [ApiController] public class DepartmentsController : ControllerBase { private readonly IDepartmentRepository departmentRepository; public DepartmentsController(IDepartmentRepository departmentRepository) { this.departmentRepository = departmentRepository; } [HttpGet] public async Task<ActionResult> GetDepartments() { try { return Ok(await departmentRepository.GetDepartments()); } catch (Exception) { return StatusCode(StatusCodes.Status500InternalServerError, "Error retrieving data from the database"); } } [HttpGet("{id:int}")] public async Task<ActionResult<Department>> GetDepartment(int id) { try { var result = await departmentRepository.GetDepartment(id); if (result == null) { return NotFound(); } return result; } catch (Exception) { return StatusCode(StatusCodes.Status500InternalServerError, "Error retrieving data from the database"); } } }
IDepartmentService Interface
public interface IDepartmentService { Task<IEnumerable<Department>> GetDepartments(); Task<Department> GetDepartment(int id); }
DepartmentService Class
public class DepartmentService : IDepartmentService { private readonly HttpClient httpClient; public DepartmentService(HttpClient httpClient) { this.httpClient = httpClient; } public async Task<Department> GetDepartment(int id) { return await httpClient.GetJsonAsync<Department>($"api/departments/{id}"); } public async Task<IEnumerable<Department>> GetDepartments() { return await httpClient.GetJsonAsync<Department[]>("api/departments"); } }
Blazor 웹 프로젝트 – Startup.cs
ConfigureServices
메서드를 사용하여 Startup
에서 AddHttpClient
메서드를 이용해 HttpClient
를 등록합니다.
public void ConfigureServices(IServiceCollection services) { services.AddRazorPages(); services.AddServerSideBlazor(); services.AddHttpClient<IEmployeeService, EmployeeService>(client => { client.BaseAddress = new Uri("https://localhost:44379/"); }); services.AddHttpClient<IDepartmentService, DepartmentService>(client => { client.BaseAddress = new Uri("https://localhost:44379/"); }); }